How to run selenium 3 tests on zalenium docker containers
By using Zalenium Docker containers for running chrome/browser drivers and selenium grid, you can run your selenium tests without the need for any dependency such as chrome, chrome driver or webdrivermanager locally.
When you use zalenium, you can view the live execution of tests through built-in vnc viewer, view test results in the dashboard with video and chromedriver logs, and control chrome instances easily through docker containers, so let’s get started first of all since selenium is dependent on docker therefore, install docker on and zalenium images on machine by running below commands-
-update Linux system
sudo apt-get update
-install docker
sudo apt install docker.io
-check docker version
sudo docker -v
-pull docker selenium and zalenium images
sudo docker pull elgalu/selenium
sudo docker pull dosel/zalenium
sudo docker images
-start zalenium, here we are starting with 2 containers with 2 chrome instances each to run 4 tests in parallel
sudo docker run --rm -dti --name zalenium -p 4444:4444 -v /var/run/docker.sock:/var/run/docker.sock -v /tmp/videos:/home/seluser/videos --privileged dosel/zalenium start --desiredContainers 2 --maxTestSessions 2
-in case something wrong happened, you can stop all containers with below command
sudo docker stop $(sudo docker ps -a -q)
-see running containers info-
sudo docker ps
-see logs of the particular container
sudo docker logs containerid
-see performance stats of containers
sudo docker stats
-check zalenium live tests panel, make sure 4444 port is accessible
http://yourserverip:4444/grid/admin/live
-check zalenium dashboard
http://yourip:4444/dashboard/#
–run selenium tests using remote web driver url of zalenium hub
in your tests“
package simplilearn.appiummarch2023;
import static org.testng.Assert.assertEquals;
import java.net.URL;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeOptions;
import org.openqa.selenium.remote.BrowserType;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.testng.annotations.Test;
public class ZaleniumTests {
@Test
public void test1() {
WebDriver driver = null;
DesiredCapabilities caps = new DesiredCapabilities();
caps.setCapability(CapabilityType.BROWSER_NAME, BrowserType.CHROME);
caps.setCapability("zal:name", "testchandan");
caps.setCapability("zal:tz", "Europe/Berlin");
caps.setCapability("zal:recordVideo", "true");
caps.setCapability("zal:screenResolution", "1920x1058");
ChromeOptions options = new ChromeOptions();
options.addArguments("disable-infobars"); // disabling infobars
options.addArguments("--disable-extensions"); // disabling extensions
options.addArguments("--disable-gpu"); // applicable to windows os only
options.addArguments("--disable-dev-shm-usage"); // overcome limited resource problems
options.addArguments("--no-sandbox"); // Bypass OS security model
options.addArguments("--headless"); // Bypass OS security model
caps.setCapability(ChromeOptions.CAPABILITY, options);
try {
driver = new RemoteWebDriver(new URL("http://yourip:4444/wd/hub"), options);
} catch (Exception e) {
e.printStackTrace();
}
driver.get("https://testautomasi.com");
try {
Thread.sleep(30000);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
assertEquals(driver.getTitle(), "Home - Welcome to automasi solutions private limited");
driver.quit();
}
}
Live Screenshots:
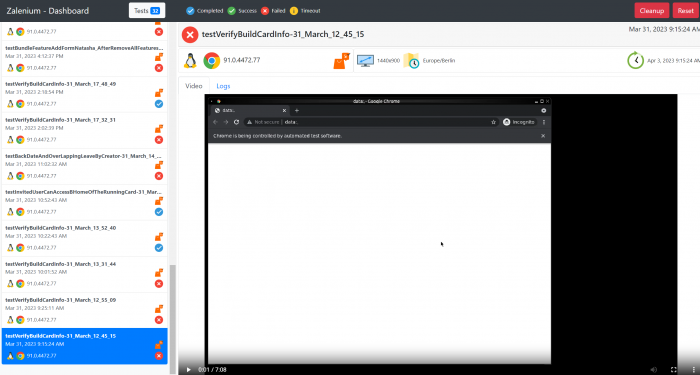
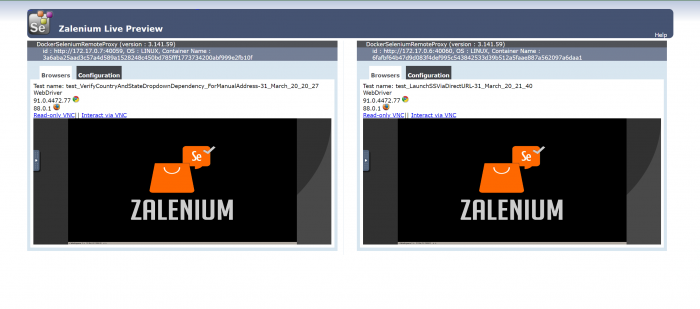
Have any feedback? Please don’t hesitate to leave it in the comments section.